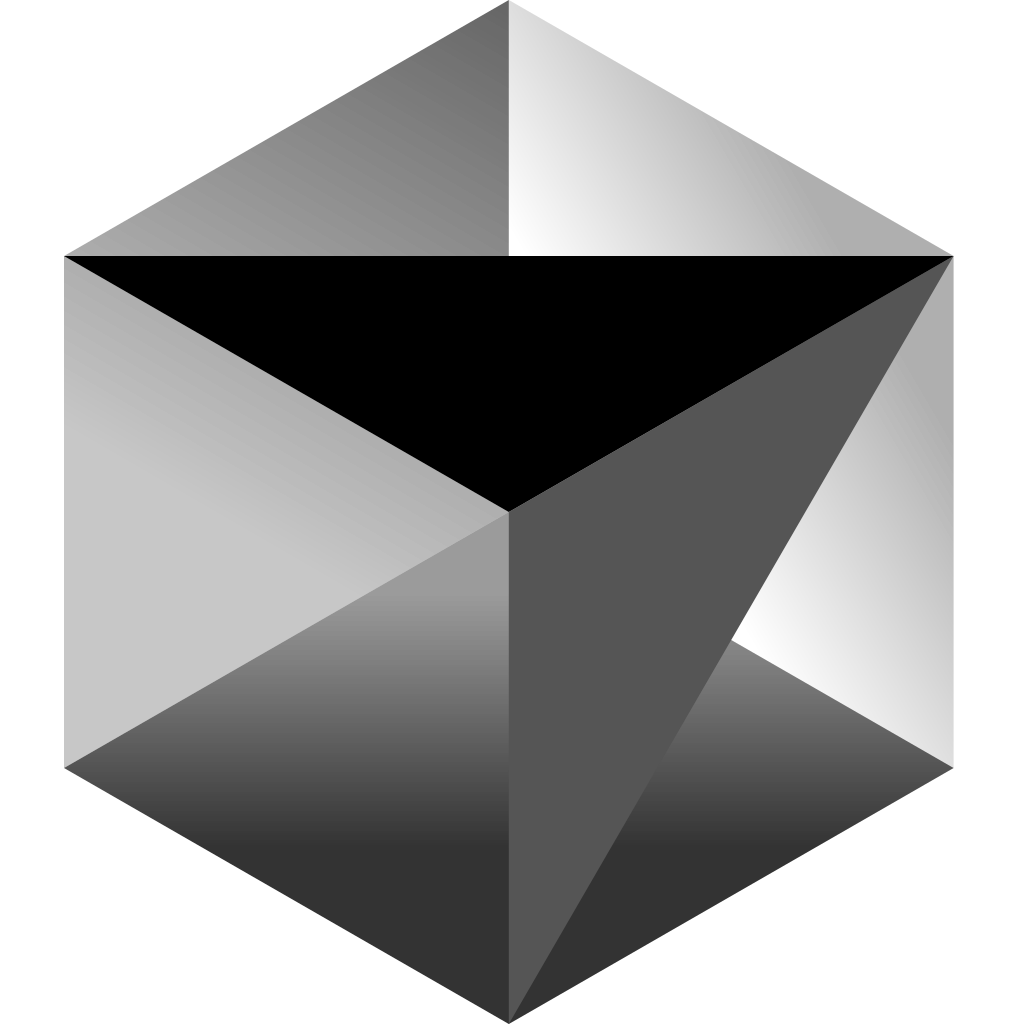
# AI-Augmented Development with Cursor, Claude, and Whispr
A practical guide to enhancing your development workflow with AI tools and voice dictation.
# Why Traditional Coding Workflows Fall Short
As developers, we've long been accustomed to writing every line of code manually, wrestling with documentation, and spending hours debugging issues that could be solved in minutes with the right guidance. Even with modern IDEs and tools, the cognitive load of translating our intentions into working code remains high, and the context-switching between writing, researching, and debugging creates significant productivity drains.
The emergence of AI coding assistants has promised to solve these problems, but many developers find themselves frustrated with inconsistent results, spending more time fighting with the AI than being helped by it. The issue isn't that AI can't help—it's that most developers haven't learned how to effectively collaborate with AI tools as part of their workflow.
This is where AI IDE tools like Cursor AI (opens new window) can shine if used smartly.
# What Makes Cursor Different
Cursor (opens new window) is an AI-enhanced code editor built on top of VSCode that goes beyond simple code completion. It offers a conversational interface that can understand context, generate entire functions, debug issues, and even explain complex code. But its true power lies in how it can be customized and guided to work according to your specific needs.
Having spent hundreds of hours working with Cursor, I've discovered that the key to unlocking its potential lies in three main areas:
- Setting up proper rules and guidelines
- Using effective prompting techniques
- Organizing your project for optimal AI collaboration
Let's explore how to master each of these elements.
# Setting Up Your Cursor Environment
The first step to effective AI collaboration is establishing clear rules that guide Cursor's behavior. This is done through special configuration files that act as instruction sets for the AI.
# The .cursorrules
File: Your AI's Instruction Manual
The .cursorrules
file serves as the primary instruction set for Cursor AI when working in your project. Here's a template to get you started:
# PROJECT OVERVIEW
- explain the projcet in a few sentences
# PERSONALITY
- you are a friendly and helpful senior full stack developer
# TECH STACK
- Frontend: React, TypeScript, Tailwind CSS
- Backend: Node.js, Express, MongoDB
- Testing: Jest, React Testing Library
# OUR .env VARIABLES
frontend/.env
backend/.env
# CURRENT FILE STRUCTURE
> run a command like `tree -L 4 -a -I 'node_modules|.git|__pycache__|.DS_Store|.pytest_cache|.vscode' | pbcopy` to get a full project tree :
src/
├── components/...
# IMPORTANT
- repeat most important steps here
- include reasoning for complex logic
# OTHER CONTEXT
..
# COMMENTS
- keep comments short
- make sure to always include comments in your code
- do not delete comments unless they are no longer needed
TIP
Update your .cursorrules
file as your project evolves. The more specific and current your rules, the more effectively Cursor can assist you.
# The .cursorignore
File: Setting Boundaries
Similar to .gitignore
, the .cursorignore
file tells Cursor which files to ignore:
.env
*.env
node_modules/
build/
dist/
*.log
This prevents Cursor from indexing sensitive or irrelevant files, keeping your AI interactions focused on what matters.
# Mastering Prompt Engineering for Cursor
The way you communicate with Cursor dramatically impacts its effectiveness. Here are the prompting techniques I've found most effective:
# Fundamental Prompting Principles
Be Specific and Detailed: Instead of asking "How do I fix this bug?", provide context: "This React component isn't re-rendering when props change. Here's the component code and how it's being used."
Set Expectations and Constraints: Add guiding principles at the end of prompts:
The fewer lines of code, the better. Proceed like a Senior Developer.
Command Completion: Reduce AI laziness with clear directives:
DO NOT STOP WORKING until you've implemented this feature fully.
# Advanced Prompting Strategies
# The Three-Paragraph Reasoning Technique
When debugging complex issues, force Cursor to think more deeply:
Start by writing 3 reasoning paragraphs analysing what the error might be. DO NOT JUMP TO CONCLUSIONS.
This prevents the AI from latching onto the first possible solution and encourages a more thorough analysis.
# The 50/50 Unbiased Evaluation
When comparing two approaches:
Before you answer, write two detailed paragraphs, one arguing for each solution. Do not jump to conclusions, seriously consider both approaches. Then, after you finish, tell me whether one solution is obviously better and why.
This counters the AI's tendency to immediately favor one approach, especially when it's trying to be agreeable.
# Perplexity Search Queries
When you need up-to-date information:
Let's perform a web search. Write a one-paragraph search query as if you were telling a human researcher what to find, including all relevant context. Format the paragraph as clear instructions, commanding a researcher to find what we're looking for.
# Voice-to-Text Integration: Supercharging Your Workflow
One of the most significant productivity boosters when working with Cursor is integrating voice-to-text tools. This approach allows you to quickly dictate instructions, code explanations, or complex queries without typing, dramatically speeding up your interaction with the AI.
# MacWhisper: Offline Transcription Power
MacWhisper (opens new window) is a powerful offline transcription tool that works exceptionally well with Cursor. As a one-time purchase application, it offers several advantages:
- Offline Processing: All transcription happens locally on your machine, ensuring privacy
- Multiple Model Options: Choose from various model sizes based on your accuracy needs
- System Prompt Integration: Customize how your dictation is processed with different system prompts
- API Flexibility: Works with both local models and cloud models (via your API tokens)
I frequently use MacWhisper to quickly dictate complex instructions to Cursor, such as explaining what I want to analyze or change in my codebase. This saves tremendous time compared to typing out detailed prompts.
# SuperWhisper: Advanced Voice Control
SuperWhisper (opens new window) takes voice dictation to the next level with more advanced features and customization options. It stands out for power users who want both dictation and AI assistant features:
- Context Awareness: Can understand context from your screen, selected text, or clipboard
- Custom Modes: Create different modes with different settings for various tasks
- Efficient Resource Management: Better system resource handling with custom model timeout
- Optional Separate Window: Expands possibilities for how you interact with the tool
The combination of SuperWhisper and Cursor creates a powerful voice-driven development environment where you can speak your intentions and watch as the AI implements them.
# Managing Context Across Sessions
When your conversation gets too long or you need to start fresh:
Before we proceed, give me a summary of the current state of the project.
Format this as 3 concise paragraphs describing what we just did, what didn't work, which files were updated/created, what mistakes to avoid, key insights/lessons learned, and problems/errors we're facing.
Write in a conversational yet informative tone, super information-dense and without fluff or noise.
DO NOT include assumptions or theories, just the facts.
# Organizing Your Project for Better AI Collaboration
How you structure your project significantly impacts Cursor's effectiveness.
# The Instructions Directory Pattern
Create an ./instructions/
directory in your project to store documentation that Cursor can reference:
instructions/
├── database_setup.md
├── api_design.md
├── component_patterns.md
└── testing_strategy.md
Each file should contain focused documentation about that aspect of your project:
# database_setup.md
## Database Structure
Our application uses MongoDB with the following collections:
- Users
- Workouts
- Exercises
## Connection Details
Connection is managed through Mongoose using environment variables:
- MONGO_URI: The connection string
- MONGO_DB_NAME: The database name
## Data Models
...
# File Headers for Context
Always include the file path at the top of your code files or instruct Cursor to do so:
// src/components/features/WorkoutTracker/ExerciseList.jsx
import React from 'react';
// ...
This helps Cursor maintain context about where each file belongs in your project structure.
# Best Practices for Efficient AI Coding
Beyond prompting and organization, these best practices will maximize your efficiency with Cursor:
# Code Quality Rules
Establish consistent rules for your code and communicate them to Cursor:
- Write clean, simple, readable code
- Implement features in the simplest possible way
- Keep files small and focused (<200 lines)
- Use clear, consistent naming
- Think thoroughly before coding
# Iterative Development Process
- Start Small: Begin with the core functionality
- Test Frequently: Verify each feature works before moving on
- Document As You Go: Add comments explaining not just what the code does, but why
# Error Handling Strategy
When encountering errors:
- DO NOT JUMP TO CONCLUSIONS! Consider multiple possible causes.
- Explain the problem in plain English first
- Make minimal necessary changes
- For strange errors, use Perplexity search for up-to-date information
# Comment Management
Comments become even more important when working with AI:
- Add helpful and explanatory comments to code
- Document changes and their reasoning in comments
- Use clear, easy-to-understand language in short sentences
- Don't delete old comments unless obviously wrong/obsolete
# Project Roadmap
Maintain a roadmap.md
file that includes:
# Current Focus
- Implementing user authentication
# Next Steps
- Add exercise database integration
- Build workout creation interface
# Log
- Tried JWT authentication but ran into issues with token persistence
- Redux implementation complete but needs performance optimization
# Priorities
- Client needs authentication completed by Friday
- Mobile responsiveness is a must-have
This helps Cursor understand your priorities and project history.
# Beyond Basic Usage: Advanced Cursor Techniques
Once you've mastered the fundamentals, these advanced techniques can further enhance your workflow:
# YOLO Mode: Empowering Agent Autonomy
One of Cursor's most powerful features when working with advanced models like Claude 3.7 Sonnet is YOLO mode. This feature allows you to configure which commands the AI agent can run automatically without requiring your confirmation.
YOLO mode is particularly valuable when running in agent mode, as it enables a more autonomous workflow while still maintaining control over critical operations:
# How to Configure YOLO Mode:
- Access Agent Settings: In Cursor, open the settings panel and navigate to the Agent configuration section
- Enable YOLO Mode: Toggle on the YOLO mode option
- Configure Command Permissions: Select which commands the agent can run without confirmation:
- Safe file operations (reading, listing directories)
- Code searches and analysis
- Simple terminal commands
- Set Restrictions: Specify which commands should always require confirmation:
- File modifications or deletions
- Installing packages or dependencies
- Network or system-critical operations
# Benefits of YOLO Mode:
- Increased Efficiency: The agent can perform routine tasks without interrupting your workflow
- Reduced Context Switching: Fewer confirmation prompts means less cognitive load
- Balanced Control: You maintain oversight of critical operations while delegating routine tasks
- Faster Development Cycles: The agent can explore, analyze, and gather information autonomously
When properly configured, YOLO mode transforms Cursor from an assistant into a true coding partner that can work alongside you, handling routine tasks while you focus on higher-level design and decision-making.
# MCP Tools: Extending Cursor's Capabilities
Model Context Protocol (MCP) (opens new window) tools extend Cursor's capabilities by connecting it to external services through a standardized protocol. MCPs essentially give Cursor new skills by allowing it to interact with specialized tools and data sources. Notable examples include the Sequential Thinking MCP (opens new window), which enables structured problem-solving through a step-by-step thinking process; the Playwright MCP (opens new window), which allows Cursor to automate browser interactions for testing and web scraping; and the Fetch MCP (opens new window), which retrieves and processes web content as markdown, even handling images. These tools transform Cursor from a code editor into a comprehensive development environment that can research, test, and interact with external resources autonomously.
# Choosing the Right Model for Your Task
The AI model you select in Cursor significantly impacts your development experience. Each model has distinct strengths that make it suitable for different coding scenarios:
Claude 3.7 Sonnet: My primary choice for most development tasks due to its excellent balance of reasoning, context understanding, and code generation. It excels at complex refactoring, debugging subtle issues, and maintaining consistency across large codebases. Its ability to follow nuanced instructions makes it particularly valuable for implementing architectural patterns.
Claude 3.5 Sonnet: Often more reliable for straightforward coding tasks and can be less prone to overthinking simple problems. It's excellent for generating boilerplate code and implementing standard patterns with minimal guidance.
O3 Mini: Perfect for quick, focused tasks where speed matters more than depth. It's ideal for generating simple utility functions, basic components, or quick syntax corrections without the overhead of larger models.
DeepSeek R1: Stands out for its specialized knowledge of programming languages and frameworks. It's my go-to for complex algorithmic problems or when working with less common libraries where other models might struggle.
Google Flash: Excels in scenarios where other models get stuck, particularly with complex mathematical reasoning or highly technical documentation. It can be a valuable alternative when you need a fresh perspective on a challenging problem.
The most important model capabilities for effective Cursor collaboration are: strong instruction following, code understanding across multiple files, reasoning about complex systems, and the ability to generate well-structured, idiomatic code in your target language. I recommend experimenting with different models for different tasks to discover which ones complement your workflow best.
# Context Syncing
For maximum efficiency, keep your mental context in sync with what Cursor knows:
- Verbalize your thought process as you work
- Summarize changes after each significant development session
- Use the instructions directory to document design decisions
# Identifying True Bottlenecks
Be honest with Cursor about your skill level and knowledge gaps:
I'm familiar with React hooks but not proficient with useCallback optimizations. Show me how to refactor this component to prevent unnecessary re-renders, with explanations for why each change helps.
# Balancing Trust with Verification
Even when Cursor sounds confident, verify important code:
- For small changes and boilerplate, trust but test
- For core business logic, verify against documentation
- For critical security features, always double-check against current best practices
# The Risks of AI-Assisted Development
While AI tools like Cursor can dramatically enhance productivity, they also introduce new risks that developers must be aware of and actively mitigate.
# Understanding the Code You Use
Perhaps the greatest risk of AI-assisted development is the temptation to implement code without fully understanding it. This creates several potential problems:
- Maintenance Challenges: When issues arise later, you may struggle to debug or modify code you don't understand
- Security Vulnerabilities: AI may generate code with subtle security flaws that aren't immediately obvious
- Technical Debt: Implementing complex solutions without understanding can lead to architectural problems as your project grows
- Skill Stagnation: Over-reliance on AI can prevent you from developing deeper technical expertise
# Trust Proportional to Impact
A crucial principle when working with AI tools is to adjust your level of scrutiny based on the importance and impact of the code:
- The bigger the decision, the less you should trust AI: For architectural decisions, security-critical code, or core business logic, use AI as a consultant rather than the decision-maker
- Verify high-impact code thoroughly: Have AI explain its reasoning, check against documentation, and consider alternative approaches
- For routine tasks, trust but verify: For boilerplate code or standard patterns, AI can be trusted more readily, but still test the results
# Developing a Healthy AI Collaboration Mindset
To use AI tools effectively while mitigating risks:
- Treat AI as a junior pair programmer: Always review its work critically
- Ask for explanations: Have the AI explain why it chose a particular approach
- Learn from the AI: Use it as a teaching tool to understand new patterns and techniques
- Maintain your expertise: Continue to deepen your understanding of fundamentals
- Establish clear boundaries: Decide which parts of your codebase require human oversight
Remember that AI tools are most valuable when they enhance your capabilities rather than replace your understanding. The goal should be to use AI to handle routine tasks while you focus on higher-level thinking, design decisions, and developing deeper expertise.
# Alternative AI Development Tools
While Cursor offers a powerful AI-enhanced development environment, several other notable tools are worth considering:
Cline (opens new window) is a free VS Code plugin that stands out for its model flexibility and cost efficiency. It supports multiple AI models (Anthropic, Gemini, DeepSeek, local LLMs) and features a unique checkpoint system for preserving environment states. Its hybrid model approach can reduce costs by up to 97% in some workflows.
Claude Code (opens new window) is Anthropic's terminal-based coding assistant that leverages the Claude 3.7 Sonnet model. It excels at analyzing large codebases (up to 200K tokens) and provides powerful features like automated refactoring, test generation, and cross-language support with impressive 92% accuracy on coding benchmarks, but is not as cost efficient.
Windsurf (opens new window) ($15/seat) brings agentic capabilities to IDE integration, featuring proactive AI assistance that analyzes multiple context signals without disrupting developer flow. Its Cascade system enables continuous workflow automation, while Supercomplete predicts and generates complete functions and documentation.
Aider (opens new window) is an open-source terminal-based coding assistant that excels in Git integration and voice coding capabilities. It supports multiple AI models and offers seamless integration with existing development workflows, though it requires CLI proficiency and careful management of API costs.
Each tool offers unique advantages: Cline's cost efficiency and model flexibility, Claude Code's deep codebase analysis, Windsurf's agentic features, and Aider's terminal-centric approach. Consider your specific needs, workflow preferences, and budget when choosing between these alternatives.
# Conclusion: The Future of AI-Enhanced Development
When used effectively, Cursor transforms from a mere code assistant into a full collaboration partner. The key is establishing clear communication patterns, organizing your project thoughtfully, and developing a workflow that combines AI strengths with human oversight.
The most successful Cursor users don't treat it as a magic solution or a perfect expert. Instead, they approach it as a powerful tool that requires proper setup and thoughtful interaction. By following the techniques outlined in this article, you can dramatically reduce the time spent on boilerplate code, debugging common issues, and researching implementation details—freeing you to focus on the creative and strategic aspects of software development.
As AI tools continue to evolve, these skills will only become more valuable. The developers who master effective AI collaboration today will have a significant advantage in the increasingly AI-augmented development landscape of tomorrow.
If you want to discuss something about optimizing your development workflow with AI tools or have questions, feel free to reach out at [email protected].